Optimizing MERN Stack Development: Best Practices and Advanced Strategies
- anujt7
- Aug 20, 2024
- 6 min read
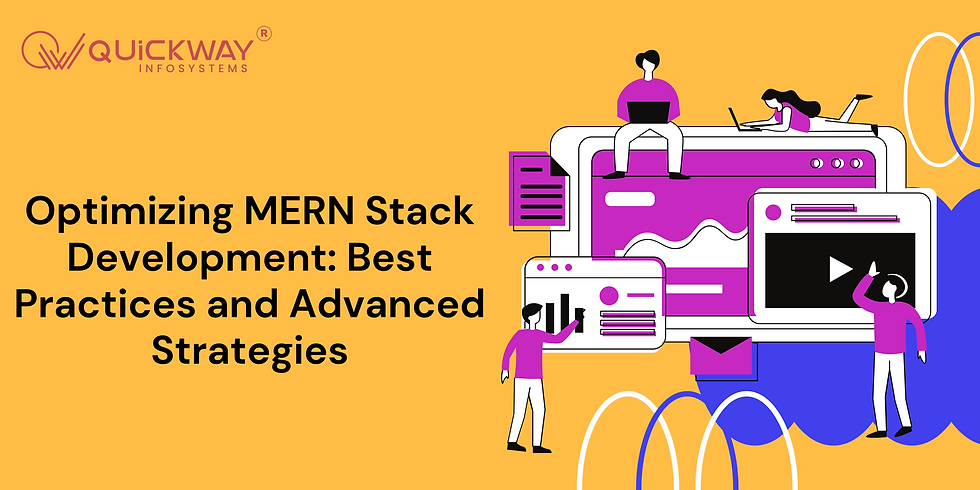
In today's fast-paced digital environment, developing applications that are efficient, scalable, and maintainable is crucial for any organization. The MERN stack, which comprises MongoDB, Express.js, React.js, and Node.js, has emerged as a popular choice for full-stack JavaScript development. However, to harness the full potential of the MERN stack, it is essential to adhere to best practices and implement advanced strategies. This article will provide in-depth insights into optimizing your MERN stack development process, focusing on the following key areas:
Architectural Design Considerations
Efficient Data Modeling and Database Management
Backend Performance Optimization
Frontend Performance Optimization
Security Best Practices
Testing and Debugging
Continuous Integration and Deployment (CI/CD)
Scalability and Monitoring
Architectural Design Considerations
Modular Code Structure
A well-organized codebase is fundamental to the success of any project. By adopting a modular architecture, developers can separate concerns and maintain a clear structure. This practice enhances code reusability, simplifies debugging, and facilitates team collaboration. For example, dividing the application into distinct modules, such as user authentication, data management, and UI components, ensures that each module can be developed, tested, and deployed independently.
Component-Based Design in React
React's component-based architecture is a powerful tool for building complex user interfaces. By breaking down the UI into reusable components, developers can create a more maintainable and scalable codebase. Components should be designed to be stateless whenever possible, with state management handled at a higher level using tools like Redux or Context API. This approach not only improves performance but also makes the application easier to test and maintain.
Efficient Data Modeling and Database Management
Schema Design in MongoDB
MongoDB's flexible schema design allows developers to store data in a way that aligns closely with the application's requirements. However, it is essential to design schemas that balance flexibility with performance. Considerations include indexing strategies, data normalization vs. denormalization, and the use of embedded documents. Proper indexing is critical for optimizing query performance, while thoughtful schema design can minimize data duplication and improve read/write operations.
Optimizing Database Operations
Efficient database operations are key to a high-performing MERN application. This involves using aggregation pipelines for complex queries, leveraging MongoDB's built-in sharding for horizontal scaling, and utilizing replica sets for high availability. Additionally, developers should regularly monitor and optimize slow queries using MongoDB's built-in performance tools.
Backend Performance Optimization
Leveraging Asynchronous Programming
Node.js is inherently asynchronous, which makes it well-suited for handling I/O-bound operations. By utilizing async/await or Promises, developers can write non-blocking code that efficiently manages multiple concurrent requests. This practice is particularly important in scenarios involving database access, file I/O, or external API calls.
Caching Strategies
Implementing effective caching strategies can significantly reduce the load on your server and improve response times. Tools like Redis or Memcached can be used to cache frequently accessed data, while HTTP caching headers can be employed to cache static assets on the client side. By reducing the frequency of expensive database queries or API calls, caching can drastically enhance the performance of your application.
Frontend Performance Optimization
Code Splitting and Lazy Loading
In React applications, code splitting and lazy loading are essential techniques for optimizing load times. By breaking down the application into smaller chunks, code splitting ensures that only the necessary code is loaded initially, reducing the initial load time. Lazy loading further enhances performance by loading components or resources only when they are needed. These techniques can be implemented using React's built-in React.lazy() and Suspense features or third-party libraries like react-loadable.
Optimizing Asset Delivery
Efficient asset delivery is crucial for a fast and responsive frontend. This includes optimizing images, using modern image formats like WebP, and employing responsive image techniques. Additionally, serving assets via a Content Delivery Network (CDN) can significantly reduce latency by delivering content from servers closer to the user. Minifying CSS and JavaScript files, as well as inlining critical CSS, can also improve load times.
Security Best Practices
Securing API Endpoints
Securing your API endpoints is critical to protecting your application from common web vulnerabilities. This includes implementing authentication and authorization mechanisms, such as JWT (JSON Web Tokens), to ensure that only authorized users can access certain resources. Additionally, input validation and sanitization are essential to prevent injection attacks, while HTTPS should be enforced to protect data in transit.
Protecting Against Cross-Site Scripting (XSS) and CSRF
Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF) are common security threats that can compromise the integrity of your application. To mitigate these risks, developers should employ Content Security Policy (CSP) headers to control the sources from which scripts can be loaded. Additionally, using secure cookies and implementing CSRF tokens can protect against unauthorized actions on behalf of authenticated users.
Testing and Debugging
Unit and Integration Testing
Thorough testing is crucial to maintaining the reliability of your MERN stack application. Unit tests should be written for individual components and functions, while integration tests ensure that different parts of the application work together as expected. Tools like Jest and Mocha can be used for unit testing, while Cypress or Selenium are popular choices for end-to-end testing.
Debugging Tools and Techniques
Effective debugging is essential for identifying and resolving issues in your application. Node.js provides built-in debugging tools like the debugger statement, while third-party tools like Nodemon and PM2 can streamline the debugging process. For frontend debugging, React Developer Tools and Redux DevTools are invaluable for inspecting component hierarchies and state changes.
Continuous Integration and Deployment (CI/CD)
Automating Build and Deployment Processes
Implementing Continuous Integration and Deployment (CI/CD) pipelines can significantly enhance the efficiency of your development process. By automating the build, testing, and deployment phases, you can ensure that code changes are reliably and consistently deployed to production. Tools like Jenkins, GitHub Actions, and CircleCI offer robust CI/CD solutions that can be integrated into your workflow.
Environment Configuration Management
Managing environment-specific configurations is critical for ensuring that your application behaves consistently across different environments (development, staging, production). Environment variables should be used to store sensitive information, and configuration files should be version-controlled and encrypted where necessary. Tools like dotenv and vault can assist in managing environment configurations securely.
Scalability and Monitoring
Horizontal Scaling Strategies
To handle increased traffic and load, horizontal scaling is often necessary. In the context of a MERN stack application, this may involve scaling your Node.js server across multiple instances or implementing a load balancer to distribute incoming requests. MongoDB's sharding feature also supports horizontal scaling by distributing data across multiple servers.
Monitoring and Logging
Continuous monitoring and logging are essential for maintaining the health and performance of your application. Tools like Prometheus, Grafana, and ELK Stack (Elasticsearch, Logstash, Kibana) provide comprehensive monitoring solutions that can track performance metrics, log errors, and visualize data in real-time. Implementing alerts and dashboards ensures that any issues can be quickly identified and addressed.
Advanced MERN Stack Optimization Techniques
Server-Side Rendering (SSR) with React
Server-Side Rendering (SSR) is an advanced technique that can significantly improve the performance and SEO of your MERN stack application. By rendering React components on the server rather than the client, you can reduce the time it takes for the initial page to load, providing a better user experience, especially on slower devices or networks. SSR can also help with SEO by ensuring that search engines can easily crawl and index your content.
Implementing SSR involves setting up your Node.js server to handle the rendering of React components. Libraries like Next.js simplify this process by providing built-in support for SSR along with other optimizations like static site generation (SSG) and automatic code splitting.
Progressive Web Apps (PWA)
Turning your MERN stack application into a Progressive Web App (PWA) can enhance the user experience by making it faster, more reliable, and capable of working offline. PWAs leverage modern web technologies like service workers, web app manifests, and caching strategies to provide a native app-like experience directly in the browser.
To implement a PWA, you need to set up a service worker to cache important assets and manage requests when the network is unavailable. Additionally, a web app manifest file allows users to add your application to their home screen, further blurring the line between web and native apps.
GraphQL for Efficient Data Fetching
While RESTful APIs are commonly used in MERN stack applications, GraphQL offers a more efficient and flexible alternative for data fetching. With GraphQL, clients can request exactly the data they need, reducing the amount of data transferred and improving performance, especially for mobile users or applications with complex data requirements.
Implementing GraphQL involves setting up a GraphQL server, typically using Apollo Server with Node.js, and defining your schema and resolvers. On the client side, Apollo Client integrates seamlessly with React, providing powerful tools for managing queries, caching, and state.
Microservices Architecture
For large-scale applications, adopting a microservices architecture can provide significant benefits in terms of scalability, maintainability, and deployment. By breaking down the application into smaller, independently deployable services, each responsible for a specific function, teams can work more autonomously and scale different parts of the application as needed.
In a MERN stack context, this might involve separating the backend into microservices for user management, payments, and notifications, each with its own database and API. Communication between microservices can be handled using HTTP, gRPC, or message brokers like RabbitMQ or Kafka.
Conclusion
Optimizing MERN stack development requires a comprehensive approach that covers everything from architectural design to performance tuning, security, and scalability. By implementing the best practices and advanced strategies outlined in this article, developers can create applications that are not only fast and reliable but also capable of evolving with the needs of the business.
These optimizations, when executed correctly, will not only improve the user experience but also position your application for better search engine rankings, contributing to the overall success of your digital presence.
Comments